View MongoDB Data
Create a data model
Run the following command from your project root to create a
Restaurant
model with name
, cuisine
, and borough
fields:
bin/rails g scaffold Restaurant name:string cuisine:string borough:string
This command also creates the controller and view files for the
Restaurant
model. You can find the directories that contain
these files in the app
directory of your application.
Retrieve specific documents
The app/controllers/restaurants_controller.rb
file contains
methods that specify how your app handles different
requests. Replace the index
method body with the following code:
def index @restaurants = Restaurant .where(name: /earth/i) end
This controller method retrieves Restaurant
documents in which the
value of the name
field contains the string "earth"
. The
results are rendered at the /restaurants
route by default.
Start your Rails application
Run the following command from the application root directory to start your Ruby web server:
bin/rails s
After the server starts, it outputs the following message
indicating that the application is running on port 3000
:
=> Booting Puma => Rails 8.0.1 application starting in development => Run `bin/rails server --help` for more startup options Puma starting in single mode... * Puma version: 6.4.3 (ruby 3.2.5-p208) ("The Eagle of Durango") * Min threads: 3 * Max threads: 3 * Environment: development * PID: 66973 * Listening on http://127.0.0.1:3000 * Listening on http://[::1]:3000 * Listening on http://127.0.2.2:3000 * Listening on http://127.0.2.3:3000 Use Ctrl-C to stop
View the restaurant data
Open the URL http://127.0.2.2:3000/restaurants in your web browser. The page shows a list of restaurants and details about each of them:
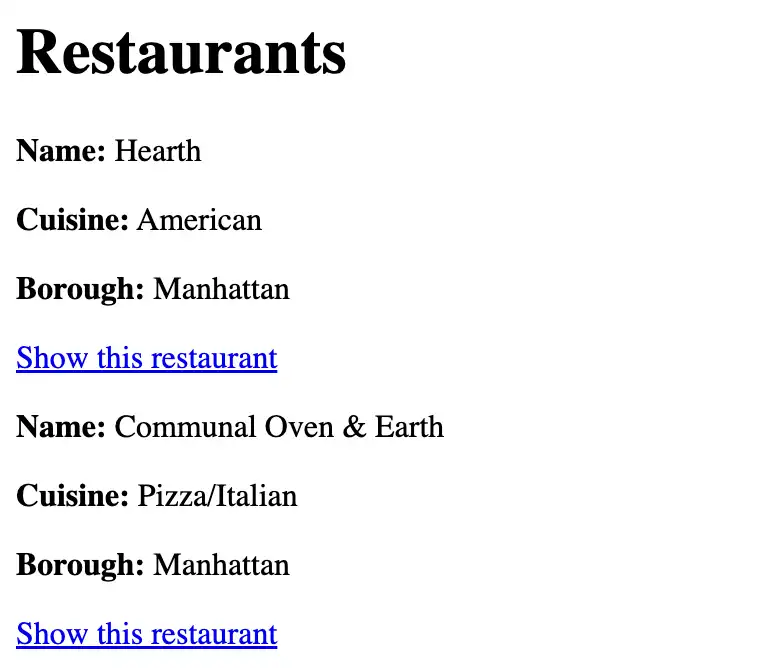
Rails provides a default interface that allows you to view, edit, and delete models. In the next section, you can learn how to use the interface to interact with MongoDB data.
Note
If you run into issues, ask for help in the MongoDB Community Forums or submit feedback by using the Feedback button in the upper right corner of the page.