Atlas Device SDK for React Native
Use Atlas Device SDK for React Native to develop iOS and Android apps with JavaScript or TypeScript.
Get Started with Realm React Native
Quick Start
Minimal-explanation code examples of how to work with the SDK. Write to the device database, and sync with other devices.
Working Example App
Learn from example by dissecting a working React Native client app that uses the React Native SDK.
Guided Tutorial
Follow a guided tutorial to learn how to adapt the example app to create your own working app.
Develop Apps with the SDK
Use the SDK's open-source database - Realm - to store data on a device. Use Device Sync to keep data in sync with your MongoDB Atlas cluster and other clients.
Install the React Native SDK
Set up your project with React Native and the SDK. To get started, install the React Native SDK.
Define an Object Schema
Use JavaScript to idiomatically define an object schema.
Configure & Open a Database
You can configure your database to do things like populate initial data on load, use an encryption key to secure data, and more. To begin working with your data, configure and open a database.
Read and Write Data
You can create, read, update, and delete objects from the device database. Construct complex queries to filter data.
React to Changes
Live objects mean that your data is always up-to-date. Register a change listener to react to changes and perform logic like updating your UI.
_Spot.webp)
Connect to an Atlas App Services App
Configure Device Sync in an App Services App. Define data access rules or use Development Mode to infer a schema from your client's data model. Then, connect to the backend App from your React Native App.
Authenticate a User
App Services provides access to custom JWT authentication, our built-in email/password provider, anonymous authentication, and popular authentication providers like Apple, Google, and Facebook. Use these providers to authenticate a user in your client.
Open a Synced Database
To get started syncing data, open a synced database. To determine what data a synced database can read and write, subscribe to a query.
Read and Write Synced Data
The APIs for reading and writing data are the same for both synced and non-synced databases. Data that you read and write to the device is automatically kept in sync with your Atlas cluster and other clients. Apps keep working offline and sync changes when a network connection is available.
_Spot.webp)
Use Atlas App Services in your React Native application with the Realm SDK.
Call Serverless Functions
To invoke serverless backend logic from your React Native client, call Atlas Functions.
Query MongoDB Atlas
Query data stored in MongoDB directly from your client application code with MongoDB Data Access.
Authenticate Users
Authenticate users with built-in and third-party authentication providers. Access App Services with authenticated users.
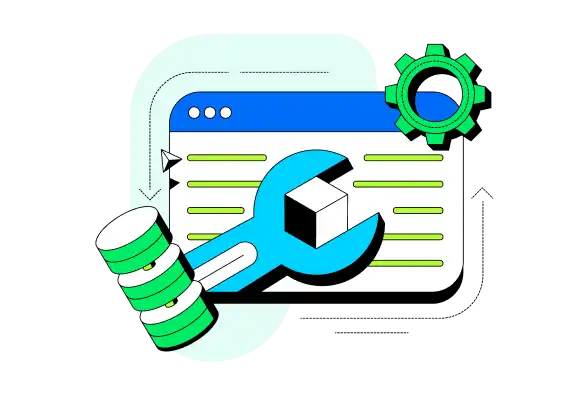
@realm/react
is an npm package that provides an easy-to-use API to
perform common SDK operations in your React Native app,
such as querying or writing to a database and listening for changes to
objects. @realm/react
includes React context, providers, and hooks
for working with the SDK.
Use @realm/react
to manage the database, Atlas App Services,
and Atlas Device Sync.
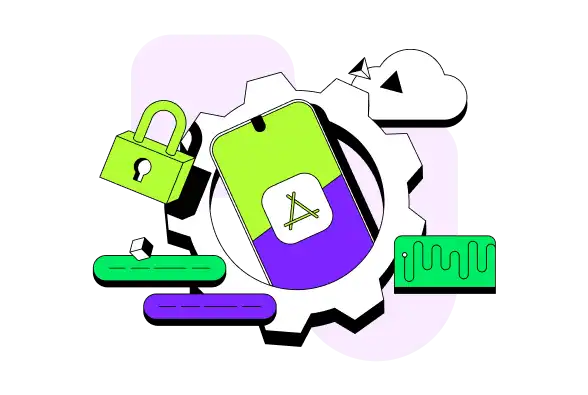
Recommended Reading
JavaScript API Reference
Explore generated reference docs for the React Native SDK.
React Native Quick Start with Expo
Build and deploy a React Native application quickly using an
Expo template application with @realm/react
.
Example Projects
Explore engineering and expert-provided example projects to learn best practices and common development patterns using the React Native SDK. Check out the Example Projects page for more React Native sample apps.
Netflix-like Movie App
Build a Netflix-like app for browsing movies from MongoDB's Mflix sample dataset. Use multiple databases to allow users to sync and manage movies in their own private lists.
Offline Login and Database Access
Log in a Device Sync user and open a synced database offline.
Connection State Change & Error Handling
Learn best practices around handling Sync errors and client reset strategies.