Getting Started with MongoDB and Java - CRUD Operations Tutorial
Rate this quickstart
- Update to Java 21
- Update Java Driver to 5.0.0
- Update
logback-classic
to 1.2.13 - Update the
preFlightChecks
method to support both MongoDB Atlas shared and dedicated clusters.
- Update to Java 17
- Update Java Driver to 4.11.1
- Update mongodb-crypt to 1.8.0
- Update Java Driver to 4.2.2.
- Added Client Side Field Level Encryption example.
- Update Java Driver to 4.1.1.
- The MongoDB Java Driver logging is now enabled via the popular SLF4J API, so I added logback in the
pom.xml
and a configuration filelogback.xml
.
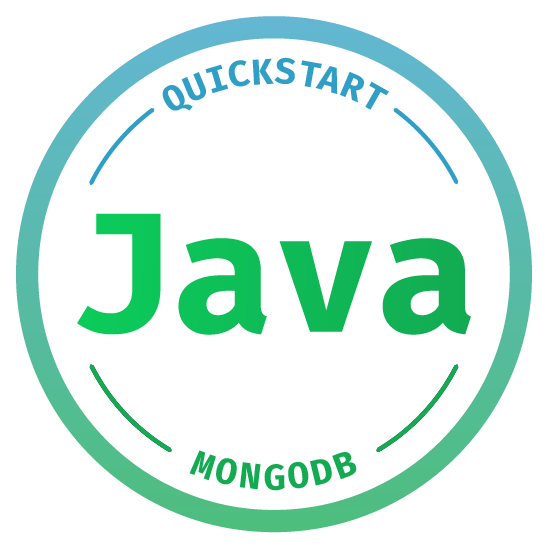
In this very first blog post of the Java Quick Start series, I will show you how to set up your Java project with Maven
and execute a MongoDB command in Java. Then, we will explore the most common operations — such as create, read, update,
and delete — using the MongoDB Java driver. I will also show you
some of the more powerful options and features available as part of the
MongoDB Java driver for each of these
operations, giving you a really great foundation of knowledge to build upon as we go through the series.
In future blog posts, we will move on and work through:
- The MongoDB Java reactive streams driver
Java is the most popular language in the IT industry at the
date of this blog post,
and developers voted MongoDB as their most wanted database four years in a row.
In this series of blog posts, I will be demonstrating how powerful these two great pieces of technology are when
combined and how you can access that power.
To follow along, you can use any environment you like and the integrated development environment of your choice. I'll
use Maven 3.8.7 and the Java OpenJDK 21, but it's fairly easy to update the code
to support older versions of Java, so feel free to use the JDK of your choice and update the Java version accordingly in
the pom.xml file we are about to set up.
For the MongoDB cluster, we will be using a M0 Free Tier MongoDB Cluster
from MongoDB Atlas. If you don't have one already, check out
my Get Started with an M0 Cluster blog post.
Get your free M0 cluster on MongoDB Atlas today. It's free forever, and you'll
be able to use it to work with the examples in this blog series.
Let's jump in and take a look at how well Java and MongoDB work together.
To begin with, we will need to set up a new Maven project. You have two options at this point. You can either clone this
series' git repository or you can create and set up the Maven project.
If you choose to use git, you will get all the code immediately. I still recommend you read through the manual set-up.
You can clone the repository if you like with the following command.
1 git clone git@github.com:mongodb-developer/java-quick-start.git
You can either use your favorite IDE to create a new Maven project for you or you can create the Maven project manually.
Either way, you should get the following folder architecture:
1 java-quick-start/ 2 ├── pom.xml 3 └── src 4 └── main 5 └── java 6 └── com 7 └── mongodb 8 └── quickstart
The pom.xml file should contain the following code:
1 2 <project xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" 3 xmlns="http://maven.apache.org/POM/4.0.0" 4 xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> 5 <modelVersion>4.0.0</modelVersion> 6 7 <groupId>com.mongodb</groupId> 8 <artifactId>java-quick-start</artifactId> 9 <version>1.0-SNAPSHOT</version> 10 11 <properties> 12 <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> 13 <maven-compiler-plugin.source>21</maven-compiler-plugin.source> 14 <maven-compiler-plugin.target>21</maven-compiler-plugin.target> 15 <maven-compiler-plugin.version>3.12.1</maven-compiler-plugin.version> 16 <mongodb-driver-sync.version>5.0.0</mongodb-driver-sync.version> 17 <mongodb-crypt.version>1.8.0</mongodb-crypt.version> 18 <!-- Keeping 1.2.13 until mongodb-crypt makes slf4j-api an optional dependency --> 19 <!-- https://jira.mongodb.org/browse/MONGOCRYPT-602 --> 20 <logback-classic.version>1.2.13</logback-classic.version> 21 <exec-maven-plugin.version>3.1.1</exec-maven-plugin.version> 22 </properties> 23 24 <dependencies> 25 <dependency> 26 <groupId>org.mongodb</groupId> 27 <artifactId>mongodb-driver-sync</artifactId> 28 <version>${mongodb-driver-sync.version}</version> 29 </dependency> 30 <dependency> 31 <groupId>org.mongodb</groupId> 32 <artifactId>mongodb-crypt</artifactId> 33 <version>${mongodb-crypt.version}</version> 34 </dependency> 35 <dependency> 36 <groupId>ch.qos.logback</groupId> 37 <artifactId>logback-classic</artifactId> 38 <version>${logback-classic.version}</version> 39 </dependency> 40 </dependencies> 41 42 <build> 43 <plugins> 44 <plugin> 45 <groupId>org.apache.maven.plugins</groupId> 46 <artifactId>maven-compiler-plugin</artifactId> 47 <version>${maven-compiler-plugin.version}</version> 48 <configuration> 49 <source>${maven-compiler-plugin.source}</source> 50 <target>${maven-compiler-plugin.target}</target> 51 </configuration> 52 </plugin> 53 <plugin> 54 <!-- Adding this plugin, so we don't need to add -Dexec.cleanupDaemonThreads=false in the mvn cmd line --> 55 <!-- to avoid the IllegalThreadStateException when running with Maven --> 56 <groupId>org.codehaus.mojo</groupId> 57 <artifactId>exec-maven-plugin</artifactId> 58 <version>${exec-maven-plugin.version}</version> 59 <configuration> 60 <cleanupDaemonThreads>false</cleanupDaemonThreads> 61 </configuration> 62 </plugin> 63 </plugins> 64 </build> 65 66 </project>
To verify that everything works correctly, you should be able to create and run a simple "Hello MongoDB!" program.
In
src/main/java/com/mongodb/quickstart
, create the HelloMongoDB.java
file:1 package com.mongodb.quickstart; 2 3 public class HelloMongoDB { 4 5 public static void main(String[] args) { 6 System.out.println("Hello MongoDB!"); 7 } 8 }
Then compile and execute it with your IDE or use the command line in the root directory (where the
src
folder is):1 mvn compile exec:java -Dexec.mainClass="com.mongodb.quickstart.HelloMongoDB"
The result should look like this:
1 [INFO] Scanning for projects... 2 [INFO] 3 [INFO] --------------------< com.mongodb:java-quick-start >-------------------- 4 [INFO] Building java-quick-start 1.0-SNAPSHOT 5 [INFO] --------------------------------[ jar ]--------------------------------- 6 [INFO] 7 [INFO] --- maven-resources-plugin:2.6:resources (default-resources) @ java-quick-start --- 8 [INFO] Using 'UTF-8' encoding to copy filtered resources. 9 [INFO] Copying 1 resource 10 [INFO] 11 [INFO] --- maven-compiler-plugin:3.12.1:compile (default-compile) @ java-quick-start --- 12 [INFO] Nothing to compile - all classes are up to date. 13 [INFO] 14 [INFO] --- exec-maven-plugin:3.1.1:java (default-cli) @ java-quick-start --- 15 Hello MongoDB! 16 [INFO] ------------------------------------------------------------------------ 17 [INFO] BUILD SUCCESS 18 [INFO] ------------------------------------------------------------------------ 19 [INFO] Total time: 0.634 s 20 [INFO] Finished at: 2024-02-19T18:12:22+01:00 21 [INFO] ------------------------------------------------------------------------
Now that our Maven project works, we have resolved our dependencies, we can start using MongoDB Atlas with Java.
If you have imported the sample dataset as suggested in
the Quick Start Atlas blog post, then with the Java code we are about
to create, you will be able to see a list of the databases in the sample dataset.
The first step is to instantiate a
MongoClient
by passing a MongoDB Atlas connection string into
the MongoClients.create()
static method. This will establish a connection
to MongoDB Atlas using the connection string. Then we can retrieve the list of
databases on this cluster and print them out to test the connection with MongoDB.As per the recommended best practices, I'm also doing a "pre-flight check" using the
{ping: 1}
admin command.In
src/main/java/com/mongodb
, create the Connection.java
file:1 package com.mongodb.quickstart; 2 3 import com.mongodb.client.MongoClient; 4 import com.mongodb.client.MongoClients; 5 import org.bson.Document; 6 import org.bson.json.JsonWriterSettings; 7 8 import java.util.ArrayList; 9 import java.util.List; 10 11 public class Connection { 12 13 public static void main(String[] args) { 14 String connectionString = System.getProperty("mongodb.uri"); 15 try (MongoClient mongoClient = MongoClients.create(connectionString)) { 16 System.out.println("=> Connection successful: " + preFlightChecks(mongoClient)); 17 System.out.println("=> Print list of databases:"); 18 List<Document> databases = mongoClient.listDatabases().into(new ArrayList<>()); 19 databases.forEach(db -> System.out.println(db.toJson())); 20 } 21 } 22 23 static boolean preFlightChecks(MongoClient mongoClient) { 24 Document pingCommand = new Document("ping", 1); 25 Document response = mongoClient.getDatabase("admin").runCommand(pingCommand); 26 System.out.println("=> Print result of the '{ping: 1}' command."); 27 System.out.println(response.toJson(JsonWriterSettings.builder().indent(true).build())); 28 return response.get("ok", Number.class).intValue() == 1; 29 } 30 }
As you can see, the MongoDB connection string is retrieved from the System Properties, so we need to set this up. Once
you have retrieved your MongoDB Atlas connection string, you
can add the
mongodb.uri
system property into your IDE. Here is my configuration with IntelliJ for example.
Or if you prefer to use Maven in command line, here is the equivalent command line you can run in the root directory:
1 mvn compile exec:java -Dexec.mainClass="com.mongodb.quickstart.Connection" -Dmongodb.uri="mongodb+srv://username:password@cluster0-abcde.mongodb.net/test?w=majority"
Note: Don't forget the double quotes around the MongoDB URI to avoid surprises from your shell.
The standard output should look like this:
1 {"name": "admin", "sizeOnDisk": 303104.0, "empty": false} 2 {"name": "config", "sizeOnDisk": 147456.0, "empty": false} 3 {"name": "local", "sizeOnDisk": 5.44731136E8, "empty": false} 4 {"name": "sample_airbnb", "sizeOnDisk": 5.761024E7, "empty": false} 5 {"name": "sample_geospatial", "sizeOnDisk": 1384448.0, "empty": false} 6 {"name": "sample_mflix", "sizeOnDisk": 4.583424E7, "empty": false} 7 {"name": "sample_supplies", "sizeOnDisk": 1339392.0, "empty": false} 8 {"name": "sample_training", "sizeOnDisk": 7.4801152E7, "empty": false} 9 {"name": "sample_weatherdata", "sizeOnDisk": 5103616.0, "empty": false}
In the Connecting with Java section, we created the classes
HelloMongoDB
and Connection
. Now we will work on
the Create
class.If you didn't set up your free cluster on MongoDB Atlas, now is great time to do so. Get the directions
for creating your cluster.
In the sample dataset, you can find the database
sample_training
, which contains a collection grades
. Each document
in this collection represents a student's grades for a particular class.1 MongoDB Enterprise Cluster0-shard-0:PRIMARY> db.grades.findOne({student_id: 0, class_id: 339}) 2 { 3 "_id" : ObjectId("56d5f7eb604eb380b0d8d8ce"), 4 "student_id" : 0, 5 "scores" : [ 6 { 7 "type" : "exam", 8 "score" : 78.40446309504266 9 }, 10 { 11 "type" : "quiz", 12 "score" : 73.36224783231339 13 }, 14 { 15 "type" : "homework", 16 "score" : 46.980982486720535 17 }, 18 { 19 "type" : "homework", 20 "score" : 76.67556138656222 21 } 22 ], 23 "class_id" : 339 24 }
And here is the extended JSON representation of the
same student. You can retrieve it in MongoDB Compass, our free GUI tool, if
you want.
Extended JSON is the human-readable version of a BSON document without loss of type information. You can read more about
the Java driver and
BSON in the MongoDB Java driver documentation.
1 { 2 "_id": { 3 "$oid": "56d5f7eb604eb380b0d8d8ce" 4 }, 5 "student_id": { 6 "$numberDouble": "0" 7 }, 8 "scores": [{ 9 "type": "exam", 10 "score": { 11 "$numberDouble": "78.40446309504266" 12 } 13 }, { 14 "type": "quiz", 15 "score": { 16 "$numberDouble": "73.36224783231339" 17 } 18 }, { 19 "type": "homework", 20 "score": { 21 "$numberDouble": "46.980982486720535" 22 } 23 }, { 24 "type": "homework", 25 "score": { 26 "$numberDouble": "76.67556138656222" 27 } 28 }], 29 "class_id": { 30 "$numberDouble": "339" 31 } 32 }
As you can see, MongoDB stores BSON documents and for each key-value pair, the BSON contains the key and the value along
with its type. This is how MongoDB knows that
class_id
is actually a double and not an integer, which is not explicit
in the mongo shell representation of this document.We have 10,000 students (
student_id
from 0 to 9999) already in this collection and each of them took 10 different
classes, which adds up to 100,000 documents in this collection. Let's say a new student (student_id
10,000) just
arrived in this university and received a bunch of (random) grades in his first class. Let's insert this new student
document using Java and the MongoDB Java driver.In this university, the
class_id
varies from 0 to 500, so I can use any random value between 0 and 500.Firstly, we need to set up our
Create
class and access this sample_training.grades
collection.1 package com.mongodb.quickstart; 2 3 import com.mongodb.client.MongoClient; 4 import com.mongodb.client.MongoClients; 5 import com.mongodb.client.MongoCollection; 6 import com.mongodb.client.MongoDatabase; 7 import org.bson.Document; 8 9 public class Create { 10 11 public static void main(String[] args) { 12 try (MongoClient mongoClient = MongoClients.create(System.getProperty("mongodb.uri"))) { 13 14 MongoDatabase sampleTrainingDB = mongoClient.getDatabase("sample_training"); 15 MongoCollection<Document> gradesCollection = sampleTrainingDB.getCollection("grades"); 16 17 } 18 } 19 }
Secondly, we need to represent this new student in Java using the
Document
class.1 Random rand = new Random(); 2 Document student = new Document("_id", new ObjectId()); 3 student.append("student_id", 10000d) 4 .append("class_id", 1d) 5 .append("scores", List.of(new Document("type", "exam").append("score", rand.nextDouble() * 100), 6 new Document("type", "quiz").append("score", rand.nextDouble() * 100), 7 new Document("type", "homework").append("score", rand.nextDouble() * 100), 8 new Document("type", "homework").append("score", rand.nextDouble() * 100)));
As you can see, we reproduced the same data model from the existing documents in this collection as we made sure
that
student_id
, class_id
, and score
are all doubles.Also, the Java driver would have generated the
_id
field with an ObjectId for us if we didn't explicitly create one
here, but it's good practice to set the _id
ourselves. This won't change our life right now, but it makes more sense
when we directly manipulate POJOs, and we want to create a clean REST API. I'm doing this in
my mapping POJOs post.Note as well that we are inserting a document into an existing collection and database, but if these didn't already
exist, MongoDB would automatically create them the first time you to go insert a document into the collection.
Finally, we can insert this document.
1 gradesCollection.insertOne(student);
Here is the final
Create
class to insert one document in MongoDB with all the details I mentioned above.1 package com.mongodb.quickstart; 2 3 import com.mongodb.client.MongoClient; 4 import com.mongodb.client.MongoClients; 5 import com.mongodb.client.MongoCollection; 6 import com.mongodb.client.MongoDatabase; 7 import org.bson.Document; 8 import org.bson.types.ObjectId; 9 10 import java.util.List; 11 import java.util.Random; 12 13 public class Create { 14 15 public static void main(String[] args) { 16 try (MongoClient mongoClient = MongoClients.create(System.getProperty("mongodb.uri"))) { 17 18 MongoDatabase sampleTrainingDB = mongoClient.getDatabase("sample_training"); 19 MongoCollection<Document> gradesCollection = sampleTrainingDB.getCollection("grades"); 20 21 Random rand = new Random(); 22 Document student = new Document("_id", new ObjectId()); 23 student.append("student_id", 10000d) 24 .append("class_id", 1d) 25 .append("scores", List.of(new Document("type", "exam").append("score", rand.nextDouble() * 100), 26 new Document("type", "quiz").append("score", rand.nextDouble() * 100), 27 new Document("type", "homework").append("score", rand.nextDouble() * 100), 28 new Document("type", "homework").append("score", rand.nextDouble() * 100))); 29 30 gradesCollection.insertOne(student); 31 } 32 } 33 }
You can execute this class with the following Maven command line in the root directory or using your IDE (see above for
more details). Don't forget the double quotes around the MongoDB URI to avoid surprises.
1 mvn compile exec:java -Dexec.mainClass="com.mongodb.quickstart.Create" -Dmongodb.uri="mongodb+srv://USERNAME:PASSWORD@cluster0-abcde.mongodb.net/test?w=majority"
1 { 2 "_id": { 3 "$oid": "5d97c375ded5651ea3462d0f" 4 }, 5 "student_id": { 6 "$numberDouble": "10000" 7 }, 8 "class_id": { 9 "$numberDouble": "1" 10 }, 11 "scores": [{ 12 "type": "exam", 13 "score": { 14 "$numberDouble": "4.615256396625178" 15 } 16 }, { 17 "type": "quiz", 18 "score": { 19 "$numberDouble": "73.06173415145801" 20 } 21 }, { 22 "type": "homework", 23 "score": { 24 "$numberDouble": "19.378205578990727" 25 } 26 }, { 27 "type": "homework", 28 "score": { 29 "$numberDouble": "82.3089189278531" 30 } 31 }] 32 }
Note that the order of the fields is different from the initial document with
"student_id": 0
.We could get exactly the same order if we wanted to by creating the document like this.
1 Random rand = new Random(); 2 Document student = new Document("_id", new ObjectId()); 3 student.append("student_id", 10000d) 4 .append("scores", List.of(new Document("type", "exam").append("score", rand.nextDouble() * 100), 5 new Document("type", "quiz").append("score", rand.nextDouble() * 100), 6 new Document("type", "homework").append("score", rand.nextDouble() * 100), 7 new Document("type", "homework").append("score", rand.nextDouble() * 100))) 8 .append("class_id", 1d);
But if you do things correctly, this should not have any impact on your code and logic as fields in JSON documents are
not ordered.
An object is an unordered set of name/value pairs.
Now that we know how to create one document, let's learn how to insert many documents.
Of course, we could just wrap the previous
insert
operation into a for
loop. Indeed, if we loop 10 times on this
method, we would send 10 insert commands to the cluster and expect 10 insert acknowledgments. As you can imagine, this
would not be very efficient as it would generate a lot more TCP communications than necessary.Instead, we want to wrap our 10 documents and send them in one call to the cluster and we want to receive only one
insert acknowledgement for the entire list.
Let's refactor the code. First, let's make the random generator a
private static final
field.1 private static final Random rand = new Random();
Let's make a grade factory method.
1 private static Document generateNewGrade(double studentId, double classId) { 2 List<Document> scores = List.of(new Document("type", "exam").append("score", rand.nextDouble() * 100), 3 new Document("type", "quiz").append("score", rand.nextDouble() * 100), 4 new Document("type", "homework").append("score", rand.nextDouble() * 100), 5 new Document("type", "homework").append("score", rand.nextDouble() * 100)); 6 return new Document("_id", new ObjectId()).append("student_id", studentId) 7 .append("class_id", classId) 8 .append("scores", scores); 9 }
And now we can use this to insert 10 documents all at once.
1 List<Document> grades = new ArrayList<>(); 2 for (double classId = 1d; classId <= 10d; classId++) { 3 grades.add(generateNewGrade(10001d, classId)); 4 } 5 6 gradesCollection.insertMany(grades, new InsertManyOptions().ordered(false));
As you can see, we are now wrapping our grade documents into a list and we are sending this list in a single call with
the
insertMany
method.By default, the
insertMany
method will insert the documents in order and stop if an error occurs during the process.
For example, if you try to insert a new document with the same _id
as an existing document, you would get
a DuplicateKeyException
.Therefore, with an ordered
insertMany
, the last documents of the list would not be inserted and the insertion process
would stop and return the appropriate exception as soon as the error occurs.As you can see here, this is not the behaviour we want because all the grades are completely independent from one to
another. So, if one of them fails, we want to process all the grades and then eventually fall back to an exception for
the ones that failed.
This is why you see the second parameter
new InsertManyOptions().ordered(false)
which is true by default.Let's refactor the code a bit and here is the final
Create
class.1 package com.mongodb.quickstart; 2 3 import com.mongodb.client.MongoClient; 4 import com.mongodb.client.MongoClients; 5 import com.mongodb.client.MongoCollection; 6 import com.mongodb.client.MongoDatabase; 7 import com.mongodb.client.model.InsertManyOptions; 8 import org.bson.Document; 9 import org.bson.types.ObjectId; 10 11 import java.util.ArrayList; 12 import java.util.List; 13 import java.util.Random; 14 15 public class Create { 16 17 private static final Random rand = new Random(); 18 19 public static void main(String[] args) { 20 try (MongoClient mongoClient = MongoClients.create(System.getProperty("mongodb.uri"))) { 21 22 MongoDatabase sampleTrainingDB = mongoClient.getDatabase("sample_training"); 23 MongoCollection<Document> gradesCollection = sampleTrainingDB.getCollection("grades"); 24 25 insertOneDocument(gradesCollection); 26 insertManyDocuments(gradesCollection); 27 } 28 } 29 30 private static void insertOneDocument(MongoCollection<Document> gradesCollection) { 31 gradesCollection.insertOne(generateNewGrade(10000d, 1d)); 32 System.out.println("One grade inserted for studentId 10000."); 33 } 34 35 private static void insertManyDocuments(MongoCollection<Document> gradesCollection) { 36 List<Document> grades = new ArrayList<>(); 37 for (double classId = 1d; classId <= 10d; classId++) { 38 grades.add(generateNewGrade(10001d, classId)); 39 } 40 41 gradesCollection.insertMany(grades, new InsertManyOptions().ordered(false)); 42 System.out.println("Ten grades inserted for studentId 10001."); 43 } 44 45 private static Document generateNewGrade(double studentId, double classId) { 46 List<Document> scores = List.of(new Document("type", "exam").append("score", rand.nextDouble() * 100), 47 new Document("type", "quiz").append("score", rand.nextDouble() * 100), 48 new Document("type", "homework").append("score", rand.nextDouble() * 100), 49 new Document("type", "homework").append("score", rand.nextDouble() * 100)); 50 return new Document("_id", new ObjectId()).append("student_id", studentId) 51 .append("class_id", classId) 52 .append("scores", scores); 53 } 54 }
As a reminder, every write operation (create, replace, update, delete) performed on a single document
is ACID in MongoDB. Which means
insertMany
is not ACID by default but, good
news, since MongoDB 4.0, we can wrap this call in a multi-document ACID transaction to make it fully ACID. I explain
this in more detail in my blog
about multi-document ACID transactions.We created the class
Create
. Now we will work in the Read
class.We wrote 11 new grades, one for the student with
{"student_id": 10000}
and 10 for the student
with {"student_id": 10001}
in the sample_training.grades
collection.As a reminder, here are the grades of the
{"student_id": 10000}
.1 MongoDB Enterprise Cluster0-shard-0:PRIMARY> db.grades.findOne({"student_id":10000}) 2 { 3 "_id" : ObjectId("5daa0e274f52b44cfea94652"), 4 "student_id" : 10000, 5 "class_id" : 1, 6 "scores" : [ 7 { 8 "type" : "exam", 9 "score" : 39.25175977753478 10 }, 11 { 12 "type" : "quiz", 13 "score" : 80.2908713167313 14 }, 15 { 16 "type" : "homework", 17 "score" : 63.5444978481843 18 }, 19 { 20 "type" : "homework", 21 "score" : 82.35202261582563 22 } 23 ] 24 }
We also discussed BSON types, and we noted that
student_id
and class_id
are doubles.MongoDB treats some types as equivalent for comparison purposes. For instance, numeric types undergo conversion before
comparison.
So, don't be surprised if I filter with an integer number and match a document which contains a double number for
example. If you want to filter documents by value types, you can use
the $type operator.
Let's read the document above. To achieve this, we will use the method
find
, passing it a filter to help identify the
document we want to find.Please create a class
Read
in the com.mongodb.quickstart
package with this code:1 package com.mongodb.quickstart; 2 3 import com.mongodb.client.*; 4 import org.bson.Document; 5 6 import java.util.ArrayList; 7 import java.util.List; 8 9 import static com.mongodb.client.model.Filters.*; 10 import static com.mongodb.client.model.Projections.*; 11 import static com.mongodb.client.model.Sorts.descending; 12 13 public class Read { 14 15 public static void main(String[] args) { 16 try (MongoClient mongoClient = MongoClients.create(System.getProperty("mongodb.uri"))) { 17 MongoDatabase sampleTrainingDB = mongoClient.getDatabase("sample_training"); 18 MongoCollection<Document> gradesCollection = sampleTrainingDB.getCollection("grades"); 19 20 // find one document with new Document 21 Document student1 = gradesCollection.find(new Document("student_id", 10000)).first(); 22 System.out.println("Student 1: " + student1.toJson()); 23 } 24 } 25 }
Also, make sure you set up your
mongodb.uri
in your system properties using your IDE if you want to run this code in
your favorite IDE.Alternatively, you can use this Maven command line in your root project (where the
src
folder is):1 mvn compile exec:java -Dexec.mainClass="com.mongodb.quickstart.Read" -Dmongodb.uri="mongodb+srv://USERNAME:PASSWORD@cluster0-abcde.mongodb.net/test?w=majority"
The standard output should be:
1 Student 1: {"_id": {"$oid": "5daa0e274f52b44cfea94652"}, 2 "student_id": 10000.0, 3 "class_id": 1.0, 4 "scores": [ 5 {"type": "exam", "score": 39.25175977753478}, 6 {"type": "quiz", "score": 80.2908713167313}, 7 {"type": "homework", "score": 63.5444978481843}, 8 {"type": "homework", "score": 82.35202261582563} 9 ] 10 }
The MongoDB driver comes with a few helpers to ease the writing of these queries. Here's an equivalent query using
the
Filters.eq()
method.1 gradesCollection.find(eq("student_id", 10000)).first();
Of course, I used a static import to make the code as compact and easy to read as possible.
1 import static com.mongodb.client.model.Filters.eq;
In the previous example, the benefit of these helpers is not obvious, but let me show you another example where I'm
searching all the grades with a student_id greater than or equal to 10,000.
1 // without helpers 2 gradesCollection.find(new Document("student_id", new Document("$gte", 10000))); 3 // with the Filters.gte() helper 4 gradesCollection.find(gte("student_id", 10000));
As you can see, I'm using the
$gte
operator to write this query. You can learn about all the
different query operators in the MongoDB documentation.The
find
method returns an object that implements the interface FindIterable
, which ultimately extends
the Iterable
interface, so we can use an iterator to go through the list of documents we are receiving from MongoDB:1 FindIterable<Document> iterable = gradesCollection.find(gte("student_id", 10000)); 2 MongoCursor<Document> cursor = iterable.iterator(); 3 System.out.println("Student list with cursor: "); 4 while (cursor.hasNext()) { 5 System.out.println(cursor.next().toJson()); 6 }
Lists are usually easier to manipulate than iterators, so we can also do this to retrieve directly
an
ArrayList<Document>
:1 List<Document> studentList = gradesCollection.find(gte("student_id", 10000)).into(new ArrayList<>()); 2 System.out.println("Student list with an ArrayList:"); 3 for (Document student : studentList) { 4 System.out.println(student.toJson()); 5 }
We could also use a
Consumer
which is a functional interface:1 Consumer<Document> printConsumer = document -> System.out.println(document.toJson()); 2 gradesCollection.find(gte("student_id", 10000)).forEach(printConsumer);
As we saw above with the
Iterator
example, MongoDB
leverages cursors to iterate through your result set.If you are already familiar with the cursors in the mongo shell, you know
that transformations can be applied to it. A cursor can
be sorted and the documents it contains can be
transformed using a projection. Also,
once the cursor is sorted, we can choose to skip a few documents and limit the number of documents in the output. This
is very useful to implement pagination in your frontend for example.
Let's combine everything we have learnt in one query:
1 List<Document> docs = gradesCollection.find(and(eq("student_id", 10001), lte("class_id", 5))) 2 .projection(fields(excludeId(), 3 include("class_id", 4 "student_id"))) 5 .sort(descending("class_id")) 6 .skip(2) 7 .limit(2) 8 .into(new ArrayList<>()); 9 10 System.out.println("Student sorted, skipped, limited and projected: "); 11 for (Document student : docs) { 12 System.out.println(student.toJson()); 13 }
Here is the output we get:
1 {"student_id": 10001.0, "class_id": 3.0} 2 {"student_id": 10001.0, "class_id": 2.0}
Remember that documents are returned in
the natural order, so if you want your output
ordered, you need to sort your cursors to make sure there is no randomness in your algorithm.
To make my last query efficient, I should create this index:
1 db.grades.createIndex({"student_id": 1, "class_id": -1})
1 "winningPlan" : { 2 "stage" : "LIMIT", 3 "limitAmount" : 2, 4 "inputStage" : { 5 "stage" : "PROJECTION_COVERED", 6 "transformBy" : { 7 "_id" : 0, 8 "class_id" : 1, 9 "student_id" : 1 10 }, 11 "inputStage" : { 12 "stage" : "SKIP", 13 "skipAmount" : 2, 14 "inputStage" : { 15 "stage" : "IXSCAN", 16 "keyPattern" : { 17 "student_id" : 1, 18 "class_id" : -1 19 }, 20 "indexName" : "student_id_1_class_id_-1", 21 "isMultiKey" : false, 22 "multiKeyPaths" : { 23 "student_id" : [ ], 24 "class_id" : [ ] 25 }, 26 "isUnique" : false, 27 "isSparse" : false, 28 "isPartial" : false, 29 "indexVersion" : 2, 30 "direction" : "forward", 31 "indexBounds" : { 32 "student_id" : [ 33 "[10001.0, 10001.0]" 34 ], 35 "class_id" : [ 36 "[5.0, -inf.0]" 37 ] 38 } 39 } 40 } 41 } 42 }
With this index, we can see that we have no SORT stage, so we are not doing a sort in memory as the documents are
already sorted "for free" and returned in the order of the index.
Also, we can see that we don't have any FETCH stage, so this is
a covered query, the most efficient type of
query you can run in MongoDB. Indeed, all the information we are returning at the end is already in the index, so the
index itself contains everything we need to answer this query.
1 package com.mongodb.quickstart; 2 3 import com.mongodb.client.*; 4 import org.bson.Document; 5 6 import java.util.ArrayList; 7 import java.util.List; 8 import java.util.function.Consumer; 9 10 import static com.mongodb.client.model.Filters.*; 11 import static com.mongodb.client.model.Projections.*; 12 import static com.mongodb.client.model.Sorts.descending; 13 14 public class Read { 15 16 public static void main(String[] args) { 17 try (MongoClient mongoClient = MongoClients.create(System.getProperty("mongodb.uri"))) { 18 MongoDatabase sampleTrainingDB = mongoClient.getDatabase("sample_training"); 19 MongoCollection<Document> gradesCollection = sampleTrainingDB.getCollection("grades"); 20 21 // find one document with new Document 22 Document student1 = gradesCollection.find(new Document("student_id", 10000)).first(); 23 System.out.println("Student 1: " + student1.toJson()); 24 25 // find one document with Filters.eq() 26 Document student2 = gradesCollection.find(eq("student_id", 10000)).first(); 27 System.out.println("Student 2: " + student2.toJson()); 28 29 // find a list of documents and iterate throw it using an iterator. 30 FindIterable<Document> iterable = gradesCollection.find(gte("student_id", 10000)); 31 MongoCursor<Document> cursor = iterable.iterator(); 32 System.out.println("Student list with a cursor: "); 33 while (cursor.hasNext()) { 34 System.out.println(cursor.next().toJson()); 35 } 36 37 // find a list of documents and use a List object instead of an iterator 38 List<Document> studentList = gradesCollection.find(gte("student_id", 10000)).into(new ArrayList<>()); 39 System.out.println("Student list with an ArrayList:"); 40 for (Document student : studentList) { 41 System.out.println(student.toJson()); 42 } 43 44 // find a list of documents and print using a consumer 45 System.out.println("Student list using a Consumer:"); 46 Consumer<Document> printConsumer = document -> System.out.println(document.toJson()); 47 gradesCollection.find(gte("student_id", 10000)).forEach(printConsumer); 48 49 // find a list of documents with sort, skip, limit and projection 50 List<Document> docs = gradesCollection.find(and(eq("student_id", 10001), lte("class_id", 5))) 51 .projection(fields(excludeId(), include("class_id", "student_id"))) 52 .sort(descending("class_id")) 53 .skip(2) 54 .limit(2) 55 .into(new ArrayList<>()); 56 57 System.out.println("Student sorted, skipped, limited and projected:"); 58 for (Document student : docs) { 59 System.out.println(student.toJson()); 60 } 61 } 62 } 63 }
Let's edit the document with
{student_id: 10000}
. To achieve this, we will use the method updateOne
.Please create a class
Update
in the com.mongodb.quickstart
package with this code:1 package com.mongodb.quickstart; 2 3 import com.mongodb.client.MongoClient; 4 import com.mongodb.client.MongoClients; 5 import com.mongodb.client.MongoCollection; 6 import com.mongodb.client.MongoDatabase; 7 import com.mongodb.client.model.FindOneAndUpdateOptions; 8 import com.mongodb.client.model.ReturnDocument; 9 import com.mongodb.client.model.UpdateOptions; 10 import com.mongodb.client.result.UpdateResult; 11 import org.bson.Document; 12 import org.bson.conversions.Bson; 13 import org.bson.json.JsonWriterSettings; 14 15 import static com.mongodb.client.model.Filters.and; 16 import static com.mongodb.client.model.Filters.eq; 17 import static com.mongodb.client.model.Updates.*; 18 19 public class Update { 20 21 public static void main(String[] args) { 22 JsonWriterSettings prettyPrint = JsonWriterSettings.builder().indent(true).build(); 23 24 try (MongoClient mongoClient = MongoClients.create(System.getProperty("mongodb.uri"))) { 25 MongoDatabase sampleTrainingDB = mongoClient.getDatabase("sample_training"); 26 MongoCollection<Document> gradesCollection = sampleTrainingDB.getCollection("grades"); 27 28 // update one document 29 Bson filter = eq("student_id", 10000); 30 Bson updateOperation = set("comment", "You should learn MongoDB!"); 31 UpdateResult updateResult = gradesCollection.updateOne(filter, updateOperation); 32 System.out.println("=> Updating the doc with {\"student_id\":10000}. Adding comment."); 33 System.out.println(gradesCollection.find(filter).first().toJson(prettyPrint)); 34 System.out.println(updateResult); 35 } 36 } 37 }
As you can see in this example, the method
updateOne
takes two parameters:- The first one is the filter that identifies the document we want to update.
- The second one is the update operation. Here, we are setting a new field
comment
with the value"You should learn MongoDB!"
.
In order to run this program, make sure you set up your
mongodb.uri
in your system properties using your IDE if you
want to run this code in your favorite IDE (see above for more details).Alternatively, you can use this Maven command line in your root project (where the
src
folder is):1 mvn compile exec:java -Dexec.mainClass="com.mongodb.quickstart.Update" -Dmongodb.uri="mongodb+srv://USERNAME:PASSWORD@cluster0-abcde.mongodb.net/test?w=majority"
The standard output should look like this:
1 => Updating the doc with {"student_id":10000}. Adding comment. 2 { 3 "_id": { 4 "$oid": "5dd5c1f351f97d4a034109ed" 5 }, 6 "student_id": 10000.0, 7 "class_id": 1.0, 8 "scores": [ 9 { 10 "type": "exam", 11 "score": 21.580800815091415 12 }, 13 { 14 "type": "quiz", 15 "score": 87.66967927111044 16 }, 17 { 18 "type": "homework", 19 "score": 96.4060480668003 20 }, 21 { 22 "type": "homework", 23 "score": 75.44966835508427 24 } 25 ], 26 "comment": "You should learn MongoDB!" 27 } 28 AcknowledgedUpdateResult{matchedCount=1, modifiedCount=1, upsertedId=null}
An upsert is a mix between an insert operation and an update one. It happens when you want to update a document,
assuming it exists, but it actually doesn't exist yet in your database.
In MongoDB, you can set an option to create this document on the fly and carry on with your update operation. This is an
upsert operation.
In this example, I want to add a comment to the grades of my student 10002 for the class 10 but this document doesn't
exist yet.
1 filter = and(eq("student_id", 10002d), eq("class_id", 10d)); 2 updateOperation = push("comments", "You will learn a lot if you read the MongoDB blog!"); 3 UpdateOptions options = new UpdateOptions().upsert(true); 4 updateResult = gradesCollection.updateOne(filter, updateOperation, options); 5 System.out.println("\n=> Upsert document with {\"student_id\":10002.0, \"class_id\": 10.0} because it doesn't exist yet."); 6 System.out.println(updateResult); 7 System.out.println(gradesCollection.find(filter).first().toJson(prettyPrint));
As you can see, I'm using the third parameter of the update operation to set the option upsert to true.
I'm also using the static method
Updates.push()
to push a new value in my array comments
which does not exist yet,
so I'm creating an array of one element in this case.This is the output we get:
1 => Upsert document with {"student_id":10002.0, "class_id": 10.0} because it doesn't exist yet. 2 AcknowledgedUpdateResult{matchedCount=0, modifiedCount=0, upsertedId=BsonObjectId{value=5ddeb7b7224ad1d5cfab3733}} 3 { 4 "_id": { 5 "$oid": "5ddeb7b7224ad1d5cfab3733" 6 }, 7 "class_id": 10.0, 8 "student_id": 10002.0, 9 "comments": [ 10 "You will learn a lot if you read the MongoDB blog!" 11 ] 12 }
The same way I was able to update one document with
updateOne()
, I can update multiple documents with updateMany()
.1 filter = eq("student_id", 10001); 2 updateResult = gradesCollection.updateMany(filter, updateOperation); 3 System.out.println("\n=> Updating all the documents with {\"student_id\":10001}."); 4 System.out.println(updateResult);
In this example, I'm using the same
updateOperation
as earlier, so I'm creating a new one element array comments
in
these 10 documents.Here is the output:
1 => Updating all the documents with {"student_id":10001}. 2 AcknowledgedUpdateResult{matchedCount=10, modifiedCount=10, upsertedId=null}
Finally, we have one last very useful method available in the MongoDB Java Driver:
findOneAndUpdate()
.In most web applications, when a user updates something, they want to see this update reflected on their web page.
Without the
findOneAndUpdate()
method, you would have to run an update operation and then fetch the document with a
find operation to make sure you are printing the latest version of this object in the web page.The
findOneAndUpdate()
method allows you to combine these two operations in one.1 // findOneAndUpdate 2 filter = eq("student_id", 10000); 3 Bson update1 = inc("x", 10); // increment x by 10. As x doesn't exist yet, x=10. 4 Bson update2 = rename("class_id", "new_class_id"); // rename variable "class_id" in "new_class_id". 5 Bson update3 = mul("scores.0.score", 2); // multiply the first score in the array by 2. 6 Bson update4 = addToSet("comments", "This comment is uniq"); // creating an array with a comment. 7 Bson update5 = addToSet("comments", "This comment is uniq"); // using addToSet so no effect. 8 Bson updates = combine(update1, update2, update3, update4, update5); 9 // returns the old version of the document before the update. 10 Document oldVersion = gradesCollection.findOneAndUpdate(filter, updates); 11 System.out.println("\n=> FindOneAndUpdate operation. Printing the old version by default:"); 12 System.out.println(oldVersion.toJson(prettyPrint)); 13 14 // but I can also request the new version 15 filter = eq("student_id", 10001); 16 FindOneAndUpdateOptions optionAfter = new FindOneAndUpdateOptions().returnDocument(ReturnDocument.AFTER); 17 Document newVersion = gradesCollection.findOneAndUpdate(filter, updates, optionAfter); 18 System.out.println("\n=> FindOneAndUpdate operation. But we can also ask for the new version of the doc:"); 19 System.out.println(newVersion.toJson(prettyPrint));
Here is the output:
1 => FindOneAndUpdate operation. Printing the old version by default: 2 { 3 "_id": { 4 "$oid": "5dd5d46544fdc35505a8271b" 5 }, 6 "student_id": 10000.0, 7 "class_id": 1.0, 8 "scores": [ 9 { 10 "type": "exam", 11 "score": 69.52994626959251 12 }, 13 { 14 "type": "quiz", 15 "score": 87.27457417188077 16 }, 17 { 18 "type": "homework", 19 "score": 83.40970667948744 20 }, 21 { 22 "type": "homework", 23 "score": 40.43663797673247 24 } 25 ], 26 "comment": "You should learn MongoDB!" 27 } 28 29 => FindOneAndUpdate operation. But we can also ask for the new version of the doc: 30 { 31 "_id": { 32 "$oid": "5dd5d46544fdc35505a82725" 33 }, 34 "student_id": 10001.0, 35 "scores": [ 36 { 37 "type": "exam", 38 "score": 138.42535412437857 39 }, 40 { 41 "type": "quiz", 42 "score": 84.66740178906916 43 }, 44 { 45 "type": "homework", 46 "score": 36.773091359279675 47 }, 48 { 49 "type": "homework", 50 "score": 14.90842128691825 51 } 52 ], 53 "comments": [ 54 "You will learn a lot if you read the MongoDB blog!", 55 "This comment is uniq" 56 ], 57 "new_class_id": 10.0, 58 "x": 10 59 }
As you can see in this example, you can choose which version of the document you want to return using the appropriate
option.
I also used this example to show you a bunch of update operators:
set
will set a value.inc
will increment a value.rename
will rename a field.mul
will multiply the value by the given number.addToSet
is similar to push but will only push the value in the array if the value doesn't exist already.
1 package com.mongodb.quickstart; 2 3 import com.mongodb.client.MongoClient; 4 import com.mongodb.client.MongoClients; 5 import com.mongodb.client.MongoCollection; 6 import com.mongodb.client.MongoDatabase; 7 import com.mongodb.client.model.FindOneAndUpdateOptions; 8 import com.mongodb.client.model.ReturnDocument; 9 import com.mongodb.client.model.UpdateOptions; 10 import com.mongodb.client.result.UpdateResult; 11 import org.bson.Document; 12 import org.bson.conversions.Bson; 13 import org.bson.json.JsonWriterSettings; 14 15 import static com.mongodb.client.model.Filters.and; 16 import static com.mongodb.client.model.Filters.eq; 17 import static com.mongodb.client.model.Updates.*; 18 19 public class Update { 20 21 public static void main(String[] args) { 22 JsonWriterSettings prettyPrint = JsonWriterSettings.builder().indent(true).build(); 23 24 try (MongoClient mongoClient = MongoClients.create(System.getProperty("mongodb.uri"))) { 25 MongoDatabase sampleTrainingDB = mongoClient.getDatabase("sample_training"); 26 MongoCollection<Document> gradesCollection = sampleTrainingDB.getCollection("grades"); 27 28 // update one document 29 Bson filter = eq("student_id", 10000); 30 Bson updateOperation = set("comment", "You should learn MongoDB!"); 31 UpdateResult updateResult = gradesCollection.updateOne(filter, updateOperation); 32 System.out.println("=> Updating the doc with {\"student_id\":10000}. Adding comment."); 33 System.out.println(gradesCollection.find(filter).first().toJson(prettyPrint)); 34 System.out.println(updateResult); 35 36 // upsert 37 filter = and(eq("student_id", 10002d), eq("class_id", 10d)); 38 updateOperation = push("comments", "You will learn a lot if you read the MongoDB blog!"); 39 UpdateOptions options = new UpdateOptions().upsert(true); 40 updateResult = gradesCollection.updateOne(filter, updateOperation, options); 41 System.out.println("\n=> Upsert document with {\"student_id\":10002.0, \"class_id\": 10.0} because it doesn't exist yet."); 42 System.out.println(updateResult); 43 System.out.println(gradesCollection.find(filter).first().toJson(prettyPrint)); 44 45 // update many documents 46 filter = eq("student_id", 10001); 47 updateResult = gradesCollection.updateMany(filter, updateOperation); 48 System.out.println("\n=> Updating all the documents with {\"student_id\":10001}."); 49 System.out.println(updateResult); 50 51 // findOneAndUpdate 52 filter = eq("student_id", 10000); 53 Bson update1 = inc("x", 10); // increment x by 10. As x doesn't exist yet, x=10. 54 Bson update2 = rename("class_id", "new_class_id"); // rename variable "class_id" in "new_class_id". 55 Bson update3 = mul("scores.0.score", 2); // multiply the first score in the array by 2. 56 Bson update4 = addToSet("comments", "This comment is uniq"); // creating an array with a comment. 57 Bson update5 = addToSet("comments", "This comment is uniq"); // using addToSet so no effect. 58 Bson updates = combine(update1, update2, update3, update4, update5); 59 // returns the old version of the document before the update. 60 Document oldVersion = gradesCollection.findOneAndUpdate(filter, updates); 61 System.out.println("\n=> FindOneAndUpdate operation. Printing the old version by default:"); 62 System.out.println(oldVersion.toJson(prettyPrint)); 63 64 // but I can also request the new version 65 filter = eq("student_id", 10001); 66 FindOneAndUpdateOptions optionAfter = new FindOneAndUpdateOptions().returnDocument(ReturnDocument.AFTER); 67 Document newVersion = gradesCollection.findOneAndUpdate(filter, updates, optionAfter); 68 System.out.println("\n=> FindOneAndUpdate operation. But we can also ask for the new version of the doc:"); 69 System.out.println(newVersion.toJson(prettyPrint)); 70 } 71 } 72 }
Let's delete the document above. To achieve this, we will use the method
deleteOne
.Please create a class
Delete
in the com.mongodb.quickstart
package with this code:1 package com.mongodb.quickstart; 2 3 import com.mongodb.client.MongoClient; 4 import com.mongodb.client.MongoClients; 5 import com.mongodb.client.MongoCollection; 6 import com.mongodb.client.MongoDatabase; 7 import com.mongodb.client.result.DeleteResult; 8 import org.bson.Document; 9 import org.bson.conversions.Bson; 10 11 import static com.mongodb.client.model.Filters.eq; 12 import static com.mongodb.client.model.Filters.gte; 13 14 public class Delete { 15 16 public static void main(String[] args) { 17 18 try (MongoClient mongoClient = MongoClients.create(System.getProperty("mongodb.uri"))) { 19 MongoDatabase sampleTrainingDB = mongoClient.getDatabase("sample_training"); 20 MongoCollection<Document> gradesCollection = sampleTrainingDB.getCollection("grades"); 21 22 // delete one document 23 Bson filter = eq("student_id", 10000); 24 DeleteResult result = gradesCollection.deleteOne(filter); 25 System.out.println(result); 26 } 27 } 28 }
As you can see in this example, the method
deleteOne
only takes one parameter: a filter, just like the find()
operation.In order to run this program, make sure you set up your
mongodb.uri
in your system properties using your IDE if you
want to run this code in your favorite IDE (see above for more details).Alternatively, you can use this Maven command line in your root project (where the
src
folder is):1 mvn compile exec:java -Dexec.mainClass="com.mongodb.quickstart.Delete" -Dmongodb.uri="mongodb+srv://USERNAME:PASSWORD@cluster0-abcde.mongodb.net/test?w=majority"
The standard output should look like this:
1 AcknowledgedDeleteResult{deletedCount=1}
Are you emotionally attached to your document and want a chance to see it one last time before it's too late? We have
what you need.
The method
findOneAndDelete()
allows you to retrieve a document and delete it in a single atomic operation.Here is how it works:
1 Bson filter = eq("student_id", 10002); 2 Document doc = gradesCollection.findOneAndDelete(filter); 3 System.out.println(doc.toJson(JsonWriterSettings.builder().indent(true).build()));
Here is the output we get:
1 { 2 "_id": { 3 "$oid": "5ddec378224ad1d5cfac02b8" 4 }, 5 "class_id": 10.0, 6 "student_id": 10002.0, 7 "comments": [ 8 "You will learn a lot if you read the MongoDB blog!" 9 ] 10 }
This time we will use
deleteMany()
instead of deleteOne()
and we will use a different filter to match more
documents.1 Bson filter = gte("student_id", 10000); 2 DeleteResult result = gradesCollection.deleteMany(filter); 3 System.out.println(result);
This is the output we get:
1 AcknowledgedDeleteResult{deletedCount=10}
Deleting all the documents from a collection will not delete the collection itself because a collection also contains
metadata like the index definitions or the chunk distribution if your collection is sharded for example.
If you want to remove the entire collection and all the metadata associated with it, then you need to use
the
drop()
method.1 gradesCollection.drop();
1 package com.mongodb.quickstart; 2 3 import com.mongodb.client.MongoClient; 4 import com.mongodb.client.MongoClients; 5 import com.mongodb.client.MongoCollection; 6 import com.mongodb.client.MongoDatabase; 7 import com.mongodb.client.result.DeleteResult; 8 import org.bson.Document; 9 import org.bson.conversions.Bson; 10 import org.bson.json.JsonWriterSettings; 11 12 import static com.mongodb.client.model.Filters.eq; 13 import static com.mongodb.client.model.Filters.gte; 14 15 public class Delete { 16 17 public static void main(String[] args) { 18 try (MongoClient mongoClient = MongoClients.create(System.getProperty("mongodb.uri"))) { 19 MongoDatabase sampleTrainingDB = mongoClient.getDatabase("sample_training"); 20 MongoCollection<Document> gradesCollection = sampleTrainingDB.getCollection("grades"); 21 22 // delete one document 23 Bson filter = eq("student_id", 10000); 24 DeleteResult result = gradesCollection.deleteOne(filter); 25 System.out.println(result); 26 27 // findOneAndDelete operation 28 filter = eq("student_id", 10002); 29 Document doc = gradesCollection.findOneAndDelete(filter); 30 System.out.println(doc.toJson(JsonWriterSettings.builder().indent(true).build())); 31 32 // delete many documents 33 filter = gte("student_id", 10000); 34 result = gradesCollection.deleteMany(filter); 35 System.out.println(result); 36 37 // delete the entire collection and its metadata (indexes, chunk metadata, etc). 38 gradesCollection.drop(); 39 } 40 } 41 }
With this blog post, we have covered all the basic operations, such as create and read, and have also seen how we can
easily use powerful functions available in the Java driver for MongoDB. You can find the links to the other blog posts
of this series just below.
If you want to learn more and deepen your knowledge faster, I recommend you check out the "MongoDB Java
Developer Path" available for free on MongoDB University.